Using .allSatisfy in Swift
You'll often find yourself in a position where you need to check if a particular condition applies across a collection of objects. Luckily, Swift provides the allSatisfy()
method to handle just that.
Let's say we want to write a function to verify that a student has completed all of the requirements for graduation.
Initially, you might write something like this:
struct Prerequisite {
var isCompleted: Bool
var courseName: String
}
struct Student {
let degreeRequirements = [
Prerequisite(isCompleted: true,
courseName: "Introduction to Programming"),
Prerequisite(isCompleted: true,
courseName: "Discrete Mathematics"),
Prerequisite(isCompleted: true,
courseName: "Mathematics for Algorithms and Systems"),
Prerequisite(isCompleted: true,
courseName: "Advanced Data Structures"),
Prerequisite(isCompleted: true,
courseName: "Theory of Computability"),
Prerequisite(isCompleted: true,
courseName: "Ubiquitous Computing")
]
func canGraduate() -> Bool {
for requirement in degreeRequirements {
if !requirement.isCompleted {
return false
}
}
return true
}
}
Or, you might get fancy and use filter
instead:
func canGraduate() -> Bool {
// Filters by failed prerequisites
// If the list is empty, the user can graduate
degreeRequirements.filter { $0.isCompleted == false }.isEmpty
}
return
keyword.While these approaches all certainly work, we can simplify this expression even further using the underutilized allSatisfy
function:
func canGraduate() -> Bool {
// Only returns true if all items in degreeRequirements
// satisfy the requirement
degreeRequirements.allSatisfy { $0.isCompleted }
}
This approach is far more readable than the previous option, and anyone reading this code can immediately see the exact requirements that will cause this function to return true
.
If you're interested in more articles about iOS Development & Swift, check out my YouTube channel or follow me on Twitter.
If you're an indie iOS developer, make sure to check out my newsletter:
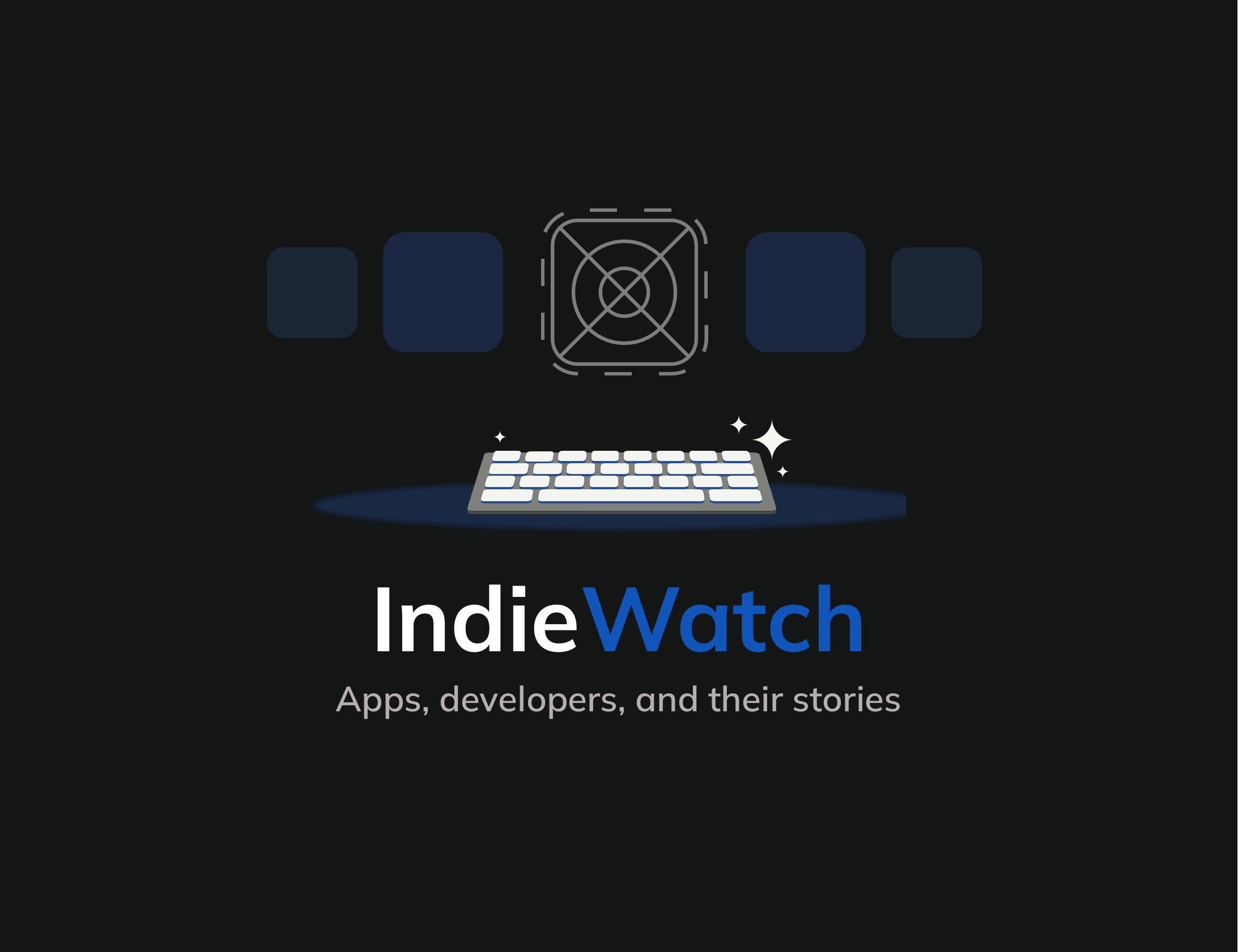
I feature a new developer every issue, so feel free to submit your indie iOS apps!
Do you have an iOS Interview coming up?
Check out my book Ace The iOS Interview!